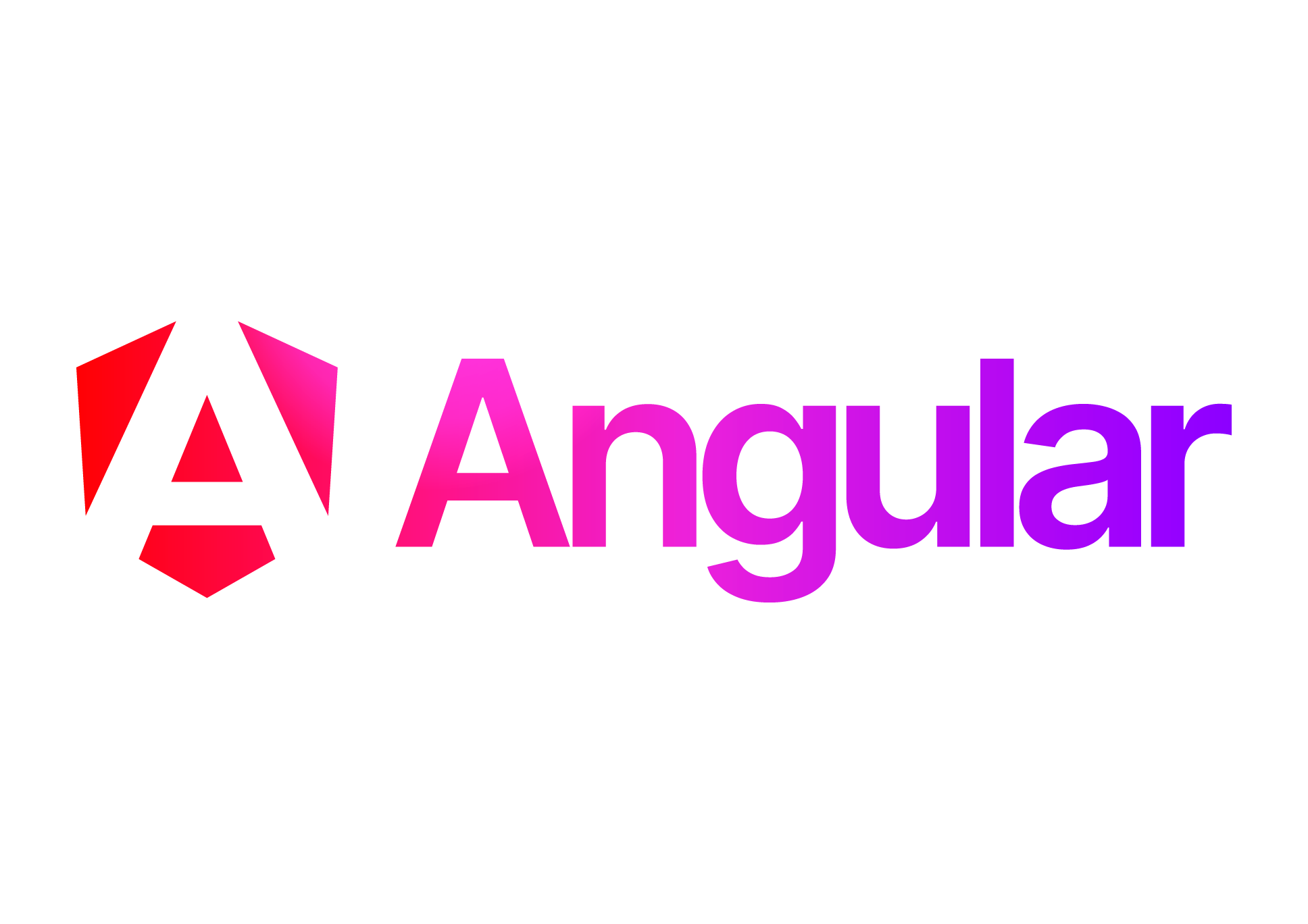
Optimizing an Angular application involves implementing various strategies to enhance performance, user experience, and reduce load times. Here, we can discuss a few techniques as follows:
Core Optimization Techniques:
Change detection: It is the process by which Angular checks if your application state has changed and if any DOM needs to be updated. Angular is implemented using Zone.js
. it is a library that essentially patches many lower-level browser APIs (like event handlers) so that the default functionality of the event occurs, as well as some custom angular functionality. Zone.js
patches all of the browser events, such as click events
, mouse events
, etc., as well as setTimeout
and setInterval
, and ajax HTTP requests. If any of these events occur in angular app, Zone.js
will trigger change detection.
OnPush
- The other change detection method that can be used in angular applications is known as the OnPush
method. With this change detection method, the component will only be checked for changes in specific scenarios.
- Input reference changes.
- An event is emitted from the component or one of its children.
- The developer has clearly indicated that the component needs to be checked.
- The async pipe is used in the view when an Observable emits an event.
If none of the above conditions are met, Angular will skip that component during its change detection cycle.
Let's clarify when change detection will run and when it won't.
this.items = [
{ id: 1, title: 'Item one' }
]
// This will not run change detection in a component
// This means that the title on the screen will remain "Item one"
this.items[0].title = 'Item one updated';
// This will run change detection, as the reference changed for this.items
const [first, ...rest] = this.items;
first.title = 'Item one updated';
this.items = [first, ...rest];
Lazy Loading: Lazy loading in Angular refers to the technique of loading website components, modules, or assets specifically when they are needed, rather than all at once. We have already discussed the lazy loading strategy.
AOT Compilation:
An Angular application is primarily comprised of components and their corresponding HTML templates. Since the components and templates provided by Angular cannot be directly understood by the browser, Angular applications need to undergo a compilation process before they are able to run in a browser.
The Angular ahead-of-time (AOT) compiler transforms Angular HTML and TypeScript code into efficient JavaScript code during the build phase, before the browser downloads and runs the code. Compiling the application during the build process results in faster rendering in the browser. AOT is the optimal compilation mode for production environments, as it reduces load time and improves performance compared to just-in-time (JIT) compilation.
Use
ng build --prod --aot
for production builds.
Tree Shaking: It refers to dead code elimination, meaning that unused modules will not be included in the bundle during the build process. While utilizing tree shaking and dead code elimination can significantly reduce the code size in an application, sending less code over the wire will improve the application's performance.
Tools like ESLint will detect dead code and mark it as "unused module," but it won't remove the code. Webpack
relies on minifiers to clean up dead code. One of them is the UglifyJS
plugin, which will eliminate the dead code from the bundle.
Bundle Size Reduction: It minimizes unused libraries and dependencies, and optimizes images and other assets. Use a tool like webpack-bundle-analyzer
to see what is causing bloat in your modules. And ensure that all the files need to be gzipped.
Server-Side Rendering (SSR): SSR apps pre-render HTML on the server, reducing the time for the browser to load content onto the screen. Use @angular/ssr
module to achieve the SSR implementation
Caching:
- Utilize browser caching for static assets.
- Explore service workers for advanced caching strategies.
These are the high-level steps to optimize the angular application.