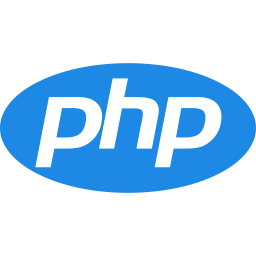
Before PHP 8.3, class constants in PHP were untyped, which could result in potential runtime errors and decreased code reliability. However, with the implementation of typed class constants in PHP 8.3, developers can now define the type of a class constant, thereby ensuring type safety and enhancing code maintainability.
<?php
class Sample {
const string TEST_CONSTANT = 'test';
}
The necessity for types in class constants is to enforce all subclasses that override class constants and not to change the type of the constants.
PHP fatal errors occur if a class constant is declared with a different type than the declaration. For example
<?php
class Sample {
const string CONST = 1;
}
In the example, you defined constants as strings and assigned them to integers, so it threw a fatal error.
Fatal error: Cannot use int as value for class constant
Sample::CONST of type string
In PHP 8.3, typed constants can be applied to the following categories:
Classes with typed constants:
<?php
class Sample {
// public constant with "string" type
const string TEST = 'test';
// protected constant with "string" type
protected const string TEST_ONE = 'test';
// final protected constant with "string" type
final protected const string TEST_TWO = 'test';
}
Traits with typed constants:
<?php
trait SampleTrait {
// final protected constant with "string" type
final protected const string FOO = 'test';
}
Interfaces with typed constants:
<?php
interface SampleInterface {
public const string FOO = 'test';
}
Enums with typed constants:
<?php
enum SampleEnum: string {
public const string FOO = 'test';
}