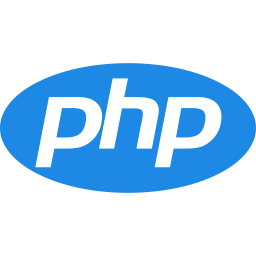
is a PHP directive "declare" that enforces strict type checking for function arguments and return values within a specific PHP file or code block, serving as a powerful tool to improve code reliability and prevent unexpected type-related issues. declare(strict_types=1)
Benefits of Using Strict Types:
- Strict typing enables early detection of potential errors during development, reducing the likelihood of runtime issues.
- Using explicit type declarations in code improves readability, making it more self-documenting and easier to understand.
- The use of strict typing enhances code maintainability by preventing unintended type-related changes when refactoring code.
- Increased Confidence: Having type-safe code can increase confidence in its correctness.
Understanding How Strict Typing Works
Without strict types, PHP performs loose type comparisons. This means that values can be implicitly converted to different types if necessary. For example, the string "5" can be treated as an integer in certain contexts.
With strict type enforcement, PHP requires precise type matching. If a function anticipates an integer and you provide a string, an error will be triggered. This compels you to be specific about data types and prevents unexpected behavior.
Example:
<?php
declare(strict_types=1);
function sum(int $a, int $b): int {
return $a + $b;
}
echo sum(1, 2);
// Output: 3
echo sum('1', 2);
// TypeError: Argument 1 passed to sum()
// must be of the type integer, string given