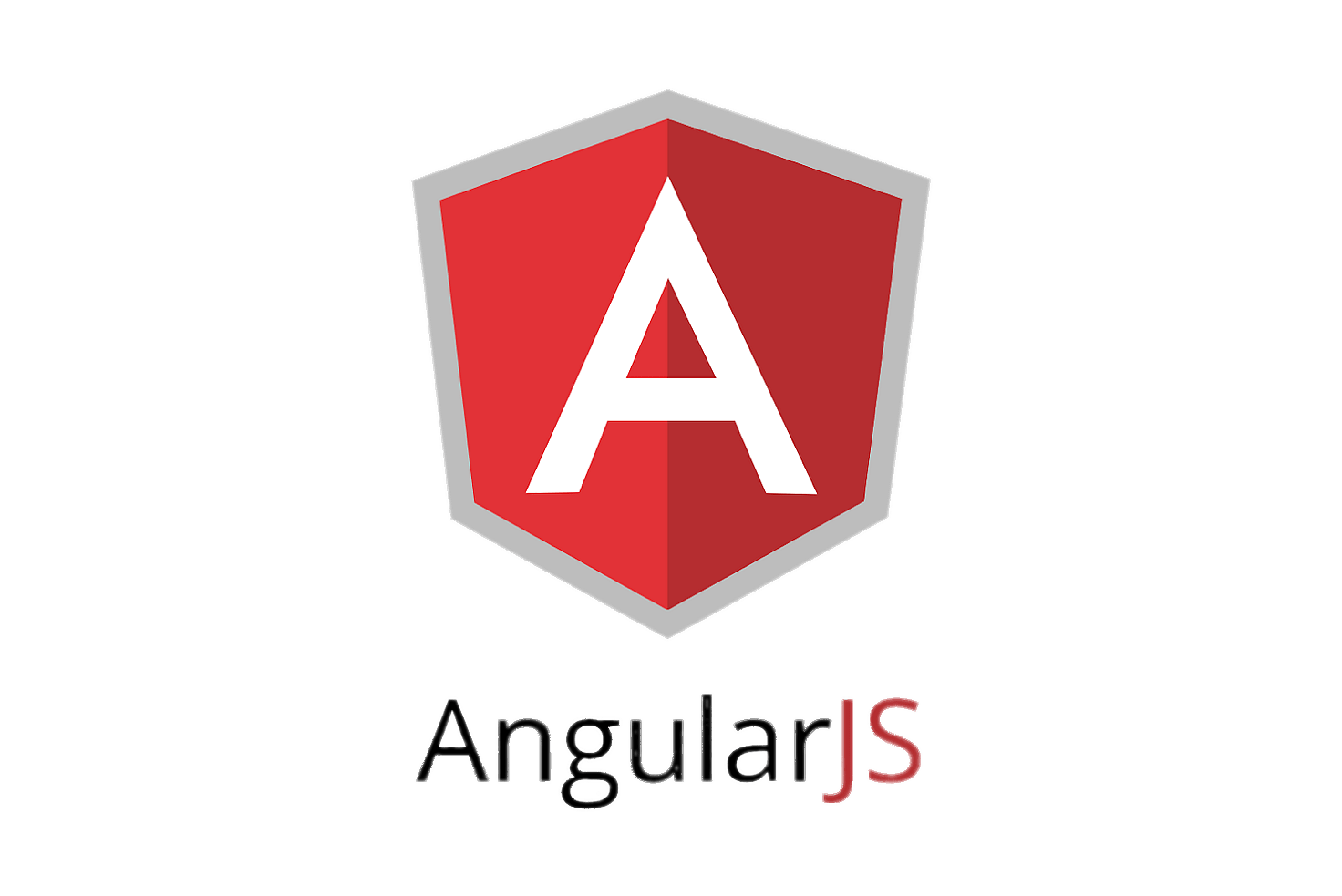
Lazy loading is a concept in Angular that enables to load modules only when they are needed, instead of loading everything at once when the application starts. This can enhance the performance of your application by reducing the initial loading time and loading only the necessary assets when required.
Benefits of using lazy loading:
- Improved initial load time: When we use lazy loading, only the modules that are needed to display the initial view of your application are loaded. This can significantly improve the initial load time of your application, especially if we have a large number of modules.
- Better performance: Lazy loading can also improve the overall performance of application by reducing the amount of memory that is used.
- Improved user experience: Lazy loading can make the application feel more responsive to users. This is because users don't have to wait for all of the modules to load before they can start using your application.
- Reduced bundle size: Lazy loading can help to reduce the size of the application's bundle, which can improve the performance of the application on mobile devices.
To implement lazy loading in Angular, we need to follow a few simple steps. First, we need to create separate modules for the components that we want to lazy load. These modules should contain the components, services, and other necessary dependencies for that part of the application.
Next, you need to set up the routes in your main app module to load these lazy modules when needed. This can be done using the loadChildren
in AppRoutingModule
property in the route configuration. For example, you can define a route like this :
const routes: Routes = [
{
path: 'user',
loadChildren: () => import('./user-module/user.module').then(
m => m.UserModule
)
}
];
This tells Angular to load the UserModule
when the 'user'
route is accessed. Angular will then lazy load the module and its dependencies only when the route is triggered, improving the performance of your application.
Lazy loading is a powerful strategy in Angular that can optimize the loading time of the application and provide a better user experience.
Full step-by-step guide : https://angular.dev/guide/ngmodules/lazy-loading#step-by-step-setup