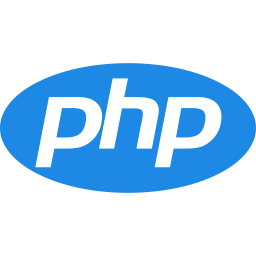
Published in
PHP
Aadhaar is a 12-digit unique identification number issued by the Indian government to residents of India. The Unique Identification Authority of India (UIDAI) is responsible for implementing and managing the Aadhaar program.
In this tutorial, we will implement Aadhaar validation using a PHP script without any API calls. I have added the AadhaarValidator class for the validation.
<?php
class AadhaarValidator
{
private static $multiplicationTable = [
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9],
[1, 2, 3, 4, 0, 6, 7, 8, 9, 5],
[2, 3, 4, 0, 1, 7, 8, 9, 5, 6],
[3, 4, 0, 1, 2, 8, 9, 5, 6, 7],
[4, 0, 1, 2, 3, 9, 5, 6, 7, 8],
[5, 9, 8, 7, 6, 0, 4, 3, 2, 1],
[6, 5, 9, 8, 7, 1, 0, 4, 3, 2],
[7, 6, 5, 9, 8, 2, 1, 0, 4, 3],
[8, 7, 6, 5, 9, 3, 2, 1, 0, 4],
[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
];
private static $permutationTable = [
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9],
[1, 5, 7, 6, 2, 8, 3, 0, 9, 4],
[5, 8, 0, 3, 7, 9, 6, 1, 4, 2],
[8, 9, 1, 6, 0, 4, 3, 5, 2, 7],
[9, 4, 5, 3, 1, 2, 6, 8, 7, 0],
[4, 2, 8, 6, 5, 7, 3, 9, 0, 1],
[2, 7, 9, 3, 8, 0, 6, 4, 1, 5],
[7, 0, 4, 6, 9, 1, 3, 2, 5, 8]
];
public static function validate(string $aadhaarNumber) : bool
{
$count = 0;
$aadhaarLength = strlen($aadhaarNumber);
$invertedAadhaarNo = array_reverse(str_split($aadhaarNumber));
foreach ($invertedAadhaarNo as $key => $value) {
$count = self::$multiplicationTable[$count][
self::$permutationTable[($key % 8)][$value]
];
}
return ($count === 0);
}
}
We can use below snippet to call the class to validate aadhaar number.
<?php
require "./AadhaarValidator.php";
$isValid = AadhaarValidator::validate('9995677770019');
var_dump($isValid);
// return bool(false)
$isValid = AadhaarValidator::validate('999999990019');
var_dump($isValid);
// return bool(true)
Useful one..